Dialog
Classic variant
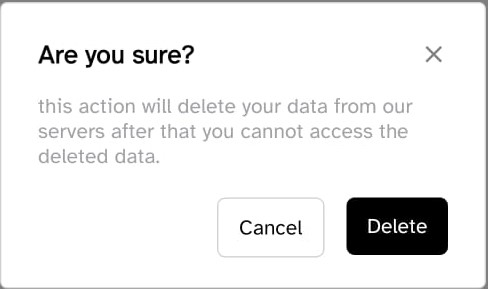
Default variant
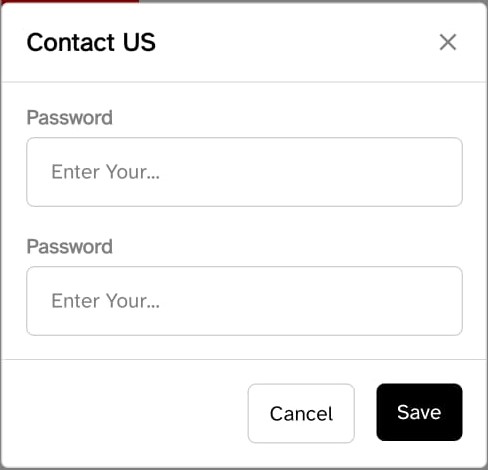
Implementation code
Wrap app.js code with PortalProvider component to use dialog.
import { PortalProvider, NativeProvider } from 'rn-nativeflow';
import Component from './path';
const App = () => {
return (
<NativeProvider>
<PortalProvider>
<Component/>
</PortalProvider>
</NativeProvider>
)
}
export default App;
- Classic variant
import { Dialog, DialogHead, DialogBody, DialogDescription, DialogFoot, Button } from 'rn-nativeflow';
const Component = () => {
return (
<Dialog isVisible={true} size='lg' variant='classic' onClose={() => {}}>
<DialogHead title='Invite your team' />
<DialogBody>
<DialogDescription>
Elevate user interactions with our versatile modals. Seamlessly integrate notifications, forms, and media displays. Make an impact effortlessly.
</DialogDescription>
</DialogBody>
<DialogFoot>
<Button
variant='outlined'
title='Cancel'
titleColor='#000'
paddingV={10}
paddingH={13}
/>
<Button
variant='contained'
title='Explore'
bg='#000'
titleColor='#fff'
paddingV={10}
paddingH={13}
/>
</DialogFoot>
</Dialog>
);
}
export default Component;
- Default variant
import { Dialog, DialogHead, DialogBody, DialogDescription, DialogFoot, Button } from 'rn-nativeflow';
const Component = () => {
return (
<Dialog isVisible={true} onClose={() => {}} >
<DialogHead title='Contact US' />
<DialogBody >
<UserInput
cursorColor='#000'
textColor='#000'
label='Password'
variant='outlined'
/>
<UserInput
cursorColor='#000'
textColor='#000'
label='Password'
variant='outlined'
/>
</DialogBody>
<DialogFoot>
<Button
variant='outlined'
title='Cancel'
titleColor='#000'
paddingV={10}
paddingH={13}
/>
<Button
variant='contained'
title='Save'
bg='#000'
titleColor='#fff'
paddingV={10}
paddingH={13}
/>
</DialogFoot>
</Dialog>
);
}
export default Component;
Props
- Dialog Props
Prop | Type | Default | Description |
---|---|---|---|
variant | classic, default | classic | It sets the variant of the dialog |
isVisible | boolean | false | It sets the visibility of the dialog |
size | xs, sm, md, lg, full | lg | It sets the horizontal size of the dialog |
fullScreen | boolean | false | Dialog cover full screen when fullScreen sets to true |
backdropColor | string | It sets the backdrop color of the dialog | |
animationDuration | number | 800 | It change the duration of closing and opening animation |
onClose | function | It fires when user click on backdrop or try to close the dialog |
- Dialog Head Props
Prop | Type | Default | Description |
---|---|---|---|
title | string | It sets the title of the dialog | |
containerStyle | string | Helps to change the styles of the dialog Head container | |
textStyle | string | Helps to change the styles of the dialog Head title |
- Dialog Body Props
Prop | Type | Default | Description |
---|---|---|---|
scrollEnable | boolean | true | It scroll the content when it overflows from the parent |
containerStyle | object | Helps to change the styles of the dialog body container |
- Dialog Description Props
Prop | Type | Default | Description |
---|---|---|---|
fs | number | 12 | It sets the font size of the description or text |
containerStyle | object | Helps to change the styles of the dialog description container | |
textStyle | object | Helps to change the styles of the dialog description text |
- Dialog Foot Props
Prop | Type | Default | Description |
---|---|---|---|
containerStyle | object | Helps to change the styles of the dialog foot container |